Python for Mobile App Development: An In-Depth Look at Popular Frameworks
- Nikhila Jain
- Mar 31, 2022
- 7 min read
Updated: Jan 27, 2024
Building mobile apps using Python can be a great way to create powerful, cross-platform applications that can run on a variety of devices. There are several libraries and frameworks available that can help you create mobile apps with Python, each with their own strengths and weaknesses.
In this blog, we will explore some of the most popular options for building mobile apps with Python and provide an in-depth look at how you can use them to create your own mobile apps.
Python
Python is a general-purpose programming language and used for different domains. It has been popular in data science and machine learning as it offers out of the box libraries.
Python language reached its popularity on desktop platforms, but with time it attained popularity in app development as well. It is flexible and uses a lot of pre-written components. In fact, it is possible to use some of the Python modules as libraries for other languages.
There are a lot of third-party packages or modules that you can import and use, including all the tools needed to do mobile development.
Kivy
Kivy is an open-source Python library for developing mobile apps. It allows developers to create multi-touch applications that run on Android, iOS, and Windows. Kivy uses its own graphics engine and supports a wide range of widgets and animations. Kivy is built on top of other open source libraries such as SDL and is designed to be easy to use and understand.
To use Kivy, you will need to install the library and its dependencies, as well as set up a development environment for your desired platform. Once you have everything set up, you can start building your app using the Kivy framework. The framework includes a wide range of widgets and UI elements that you can use to build your app, such as buttons, labels, and text inputs. You can also use Kivy's built-in animations and transitions to create a polished and professional-looking app.
One of the main advantages of using Kivy is that it allows you to create apps that run on multiple platforms with minimal changes to your code. This means that you can write your app once and then deploy it to multiple platforms, which can save you a lot of time and effort.
Another advantage of Kivy is that it is built on top of other open source libraries, which means that you can easily access the full power of those libraries within your app. For example, you can use Kivy to create apps that use the camera or GPS on a device, or that access the device's storage.
BeeWare
BeeWare is a collection of tools for building mobile apps in Python. The BeeWare suite includes Toga, a cross-platform native GUI toolkit, and Batavia, a JavaScript interpreter for Python bytecode. With BeeWare, you can write your app in Python and then use tools like Toga to create native user interfaces for different platforms.
Toga is the main tool in BeeWare for creating mobile apps. It is a cross-platform native GUI toolkit that allows you to create apps that look and feel like native apps on different platforms. Toga includes a wide range of widgets and UI elements that you can use to build your app, such as buttons, labels, and text inputs. You can also use Toga's built-in animations and transitions to create a polished and professional-looking app.
Another tool in BeeWare is Batavia, a JavaScript interpreter for Python bytecode. This tool allows you to run Python code in a JavaScript environment, which can be useful if you want to create apps that run on web browsers.
One of the main advantages of using BeeWare is that it allows you to create apps that look and feel like native apps on different platforms. This means that you can create apps that are well-suited to the platform they are running on, which can help to improve the user experience.
Another advantage of BeeWare is that it allows you to write your app in Python and then use tools like Toga to create native user interfaces for different platforms. This can help to save you time and effort, as you only have to write your app once and then deploy it to multiple platforms.
PyMob
PyMob is a platform for building mobile apps using Python. It allows developers to write their app logic in Python and then use a tool called PyMobGen to generate the user interface and other platform-specific code. PyMob supports both Android and iOS platforms.
PyMobGen is a code generation tool that takes your Python code and generates the necessary platform-specific code to build your app. This means that you can write your app logic in Python and then use PyMobGen to generate the user interface and other platform-specific code. This can save you a lot of time and effort, as you only have to write your app once and then use PyMobGen to generate the code for different platforms.
One of the main advantages of using PyMob is that it allows you to write your app logic in Python and then use a code generation tool to create the user interface and other platform-specific code. This can help to save you time and effort, as you only have to write your app once and then use PyMobGen to generate the code for different platforms.
Another advantage of PyMob is that it supports both Android and iOS platforms, which means that you can create apps that run on a wide range of devices.
Flask
Flask is a popular micro web framework for Python. It can be used to build web apps that can be accessed via a browser, but it can also be used to create mobile apps using a concept called "Progressive Web Apps" (PWAs) which are web apps that can be installed on a user's home screen and run offline.
PWAs are web apps that are designed to look and feel like native apps, but they are actually just web pages that are accessed via a browser. PWAs can be installed on a user's home screen and run offline, which makes them a great option for creating mobile apps.
One of the main advantages of using Flask to create PWAs is that it allows you to create apps that are accessible via a browser. This means that you can create apps that can be accessed from any device with a browser, which can help to increase the reach of your app.
Another advantage of using Flask to create PWAs is that it allows you to create apps that can run offline. This can be useful if you want to create apps that are designed to be used in areas with poor internet connectivity, such as remote areas or on airplanes.
QPython
QPython is an app that allows you to run Python code on Android devices. It includes a Python interpreter, as well as a library of modules. With QPython, you can write Python code on your Android device and run it directly.
One of the main advantages of using QPython is that it allows you to write Python code on your Android device and run it directly. This can be useful if you want to create apps that are designed to be used on Android devices, as you can write and test your code on the same device that your app will be running on.
Another advantage of using QPython is that it includes a library of modules, which means that you can use pre-built code to add functionality to your app.
Pyqt
PyQt is a set of Python bindings for the Qt application framework and runs on all platforms supported by Qt including Windows, OS X, Linux, iOS, and Android. PyQt5 allows you to use the Qt libraries to create desktop, mobile, and web apps with Python bindings.
One of the main advantages of using PyQt is that it allows you to create apps that run on all platforms supported by Qt, which includes Windows, OS X, Linux, iOS, and Android.
This means that you can write your app once and then deploy it to multiple platforms, which can save you a lot of time and effort.
Another advantage of PyQt is that it allows you to use the Qt libraries to create your app. The Qt libraries are a powerful set of tools that can help you create professional-looking, feature-rich apps. With PyQt, you can use the same libraries to create desktop, mobile, and web apps, which can help to streamline the development process.
Additionally, PyQt is a mature and stable framework, with a large and active community of developers who can provide support and resources. This makes it a great option for creating apps that require a lot of features and functionalities.
Here's an example mobile app that is created using Kivy.
Build Mobile App using Kivy
To create a simple app using kivy you can follow these steps:
Install kivy library by running
pip install kivy
Create a new python file and import kivy
Create a class that inherits from kivy's App class
Create a build method that returns a widget
Create a run method that starts the app
import kivy
from kivy.app import App
from kivy.uix.button import Button
class MyApp(App):
def build(self):
return Button(text='Hello World')
if __name__ == '__main__':
MyApp().run()
This is a simple example of how you can create a "Hello World" button using kivy. For more information, see more examples and tutorials on kivy's official website.
When you execute your above code sample, you will see the output generated as below:
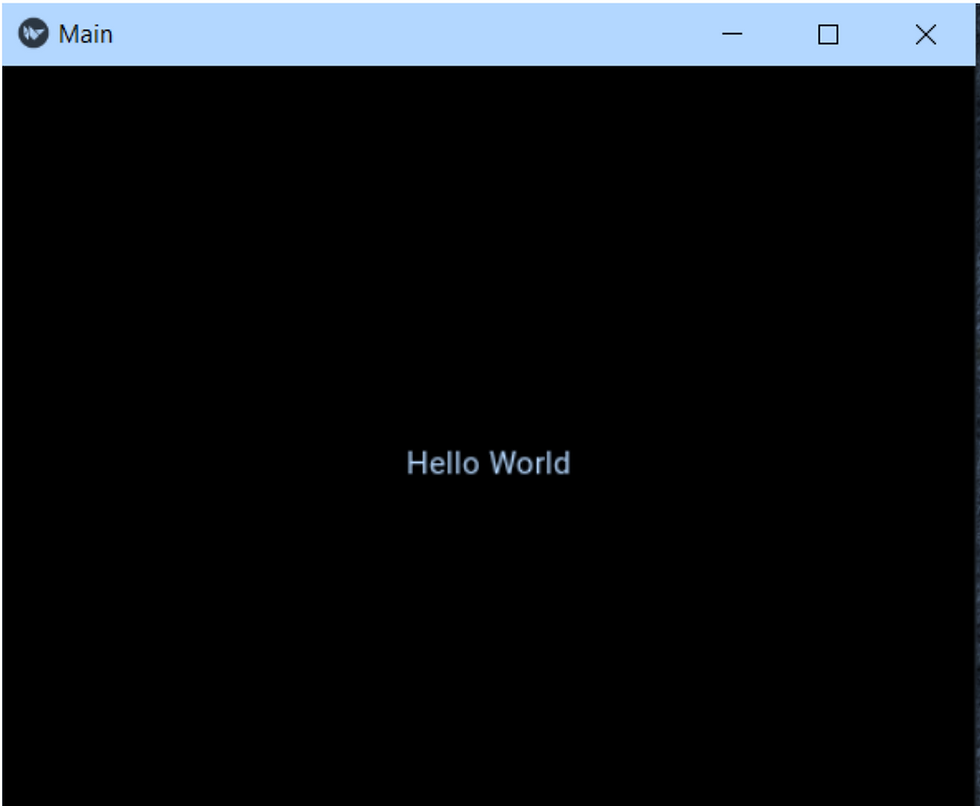
It is important to note that creating a mobile app is a complex task that requires knowledge of various programming concepts, libraries, and frameworks. It also requires a good understanding of mobile development best practices and how to design user-friendly interfaces.
Some of the use cases which are best suited for development using Python are:
Command-line utility apps
Complex apps like analytical tools that can operate on huge datasets, Banking mobile apps, Trading apps, and more.
Game development
System administrative apps
Take away
Building mobile apps using Python is possible with the help of various libraries and frameworks like Kivy, BeeWare, PyMob, Flask, QPython, Pyqt etc. Each have their own pros and cons and it's up to the developer to decide which one to use based on their specific needs and requirements. Whether you want to create a simple app that runs on multiple platforms or a more complex app that takes advantage of the latest mobile technologies, there is a Python library or framework that can help you achieve your goals.
With the right tools and a bit of creativity, you can create mobile apps that are powerful, polished, and user-friendly.
Nice intro survey. Thanks